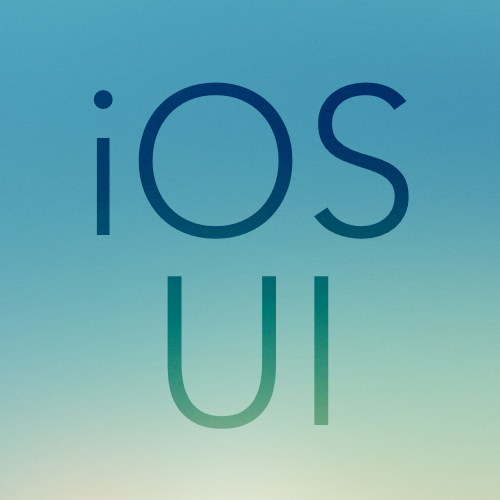
iOSでマテリアルデザインのようなタブが作れるHMSegmentedControl
今回はSegmentedControlがタブのような見た目になるOSSをご紹介します。 タブ自体のデザインがマテリアルデザインっぽいので *1、 iOSとAndroid、両方リリースするようなアプリで使えるのかなと思います。(感想)
下記画像はTabs - Components - Google design guidelinesppからお借りしたものです。
こんな感じの雰囲気を目指してみます。
環境
今回は下記で試してます。
項目 | バージョン |
---|---|
XCODE | 6.3.2 |
iOS SDK | 8.0 |
HMSegmentedControl | v1.5.1 |
また、Objectice-cでコードを書いてます。
インストール方法
新規でプロジェクトを作った後、(プロジェクトテンプレートはSingleViewApplicationでOKです) CocoaPods で
pod 'HMSegmentedControl'
pod installでインストール完了です。
実装サンプル
Storyboard
Storyboardは以下のような感じで配置してみました。赤い枠で囲っている部分に'HMSegmentedControl'を追加します。 'HMSegmentedControl'はコード上で追加するため、配置場所の確保な感じになります。
code
Storyboard上に配置したタブを配置するViewとScrollViewをOutletで接続しておきます。
#import "ViewController.h" #import "HMSegmentedControl.h" @interface ViewController () <UIScrollViewDelegate> // 画面上部のView @property (weak, nonatomic) IBOutlet UIView *headerView; // スクロールエリア @property (weak, nonatomic) IBOutlet UIScrollView *scrollView; // タブ型segmentedControl @property (nonatomic, strong) HMSegmentedControl *segmentedControl; @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; CGFloat scrollWidth = self.view.frame.size.width; CGFloat scrollHeight = self.view.frame.size.height - self.headerView.frame.size.height; // 画面上部のViewのサイズにあわせて生成する self.segmentedControl = [[HMSegmentedControl alloc] initWithFrame:CGRectMake(0, 0, self.view.frame.size.width, self.headerView.frame.size.height)]; // タブのタイトルを設定 self.segmentedControl.sectionTitles = @[@"ITEM ONE",@"ITEM TWO",@"ITEM THREE"]; // 最初の選択状態を設定 self.segmentedControl.selectedSegmentIndex = 0; // 非選択状態の背景色を設定 self.segmentedControl.backgroundColor = [UIColor colorWithRed:0.15 green:0.69 blue:0.79 alpha:1.0]; // 非選択状態のタイトル文字装飾を設定 self.segmentedControl.titleTextAttributes = @{NSForegroundColorAttributeName : [UIColor colorWithRed:0.64 green:0.91 blue:0.93 alpha:1.0]}; // 選択状態のタイトル文字装飾を設定 self.segmentedControl.selectedTitleTextAttributes = @{NSForegroundColorAttributeName : [UIColor whiteColor]}; // Indicatorの色を設定 self.segmentedControl.selectionIndicatorColor = [UIColor colorWithRed:1.00 green:1.00 blue:0.24 alpha:0.5]; // 選択状態のスタイルを設定 self.segmentedControl.selectionStyle = HMSegmentedControlSelectionStyleBox; // Indicatorの位置を設定 self.segmentedControl.selectionIndicatorLocation = HMSegmentedControlSelectionIndicatorLocationDown; __weak typeof(self) weakSelf = self; [self.segmentedControl setIndexChangeBlock:^(NSInteger index) { [weakSelf.scrollView scrollRectToVisible:CGRectMake(scrollWidth * index, 0, scrollWidth, 200) animated:YES]; }]; [self.headerView addSubview:self.segmentedControl]; // スクロール用のコンテンツを生成(色付きのViewを作成する) self.scrollView.pagingEnabled = YES; self.scrollView.showsHorizontalScrollIndicator = NO; self.scrollView.contentSize = CGSizeMake(scrollWidth * 3, scrollHeight); self.scrollView.delegate = self; UIView *page1view = [[UIView alloc] initWithFrame:CGRectMake(0, 0, scrollWidth, scrollHeight)]; page1view.backgroundColor = [UIColor colorWithRed:0.94 green:0.38 blue:0.57 alpha:1.0]; [self.scrollView addSubview:page1view]; UIView *page2view = [[UIView alloc] initWithFrame:CGRectMake(scrollWidth, 0, scrollWidth, scrollHeight)]; page2view.backgroundColor = [UIColor colorWithRed:0.47 green:0.53 blue:0.80 alpha:1.0]; [self.scrollView addSubview:page2view]; UIView *page3view = [[UIView alloc] initWithFrame:CGRectMake(scrollWidth * 2, 0, scrollWidth, scrollHeight)]; page3view.backgroundColor = [UIColor colorWithRed:1.00 green:0.72 blue:0.30 alpha:1.0]; [self.scrollView addSubview:page3view]; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; } @end
プロパティ
主に使われそうなものをまとめてみました。
プロパティ名 | 型 | 説明 |
---|---|---|
type | HMSegmentedControlType | 'テキスト'or'画像'or'テキストと画像'を設定する |
sectionTitles | NSArray | タブのタイトルを配列で設定する |
selectedSegmentIndex | NSInteger | タブ選択のindex |
backgroundColor | UIColor | タブの背景色 |
titleTextAttributes | NSDictionary | 非選択状態のタイトル文字装飾(NSAttributedString) |
selectedTitleTextAttributes | NSDictionary | 選択状態のタイトル文字装飾(NSAttributedString) |
selectionIndicatorColor | UIColor | Indicatorの色 |
segmentWidthStyle | HMSegmentedControlSegmentWidthStyle | 横幅のスタイル。(固定or文字の長さ) |
selectionStyle | HMSegmentedControlSelectionStyle | 選択状態のスタイル |
selectionIndicatorLocation | HMSegmentedControlSelectionIndicatorLocation | Indicatorの位置 |
selectionStyle
選択状態のスタイルは以下から選択します。
HMSegmentedControlSelectionStyleTextWidthStripe | 文字の長さ | ![]() |
---|---|---|
HMSegmentedControlSelectionStyleFullWidthStripe | タブの長さ | ![]() |
HMSegmentedControlSelectionStyleBox | Box型 | ![]() |
HMSegmentedControlSelectionStyleArrow | さんかく | ![]() |
selectionIndicatorLocation
Indicatorの位置は以下から選択します
HMSegmentedControlSelectionIndicatorLocationUp | 上 | ![]() |
---|---|---|
HMSegmentedControlSelectionIndicatorLocationDown | 下 | ![]() |
HMSegmentedControlSelectionIndicatorLocationNone | 無し | ![]() |
実行結果
シミュレーターで実行してみました。
脚注
- 個人的な主観です ↩